Higher-Order Components, and how to use them?
According to the React official documentation, HOCs (Higher-order component) is an advanced technique in React for reusing component logic. Wooh, that sounds scary, doesn’t it? In today’s world, a lot of official programming language documentation and guides actually talk about topics using really complicated and fancy words, so even such simple techniques might seem challenging to understand.
But in reality, HOCs are fun and have many uses that are not always obvious to developers. They can prepare properties, manage the state, or alter the component representation. Still scary? Let’s have a closer look!
HOCs basically incorporate the don’t-repeat-yourself (DRY) principle of programming, which you have most likely come across at some point in your career as a software developer. Some HOC use cases include:
- Infinite scroll in three different views, all having different data
- Components using data from third party subscription
- App components that need logged in user data
- Showing multiple lists(e.g. Users, Locations) with search feature
- Enhancing different card views with same border and shadow
HOCs are not part of the React API, as they are a pattern that emerges from React’s compositional nature. HOCs can take any input data, which most often is a component and some other optional arguments, and return a new component as output. The HOC returned component is an improved input component that can now be used in JSX.
Higher-Order Functions In JavaScript
Before jumping into React Higher-Order Components, let’s briefly discuss higher-order functions in JavaScript. Understanding this is critical to understanding our topic of focus.
Higher-order functions in JavaScript take some functions as arguments and return another function. They enable developers to abstract over actions, not just values, and they help us to write less code when operating on functions and even arrays.
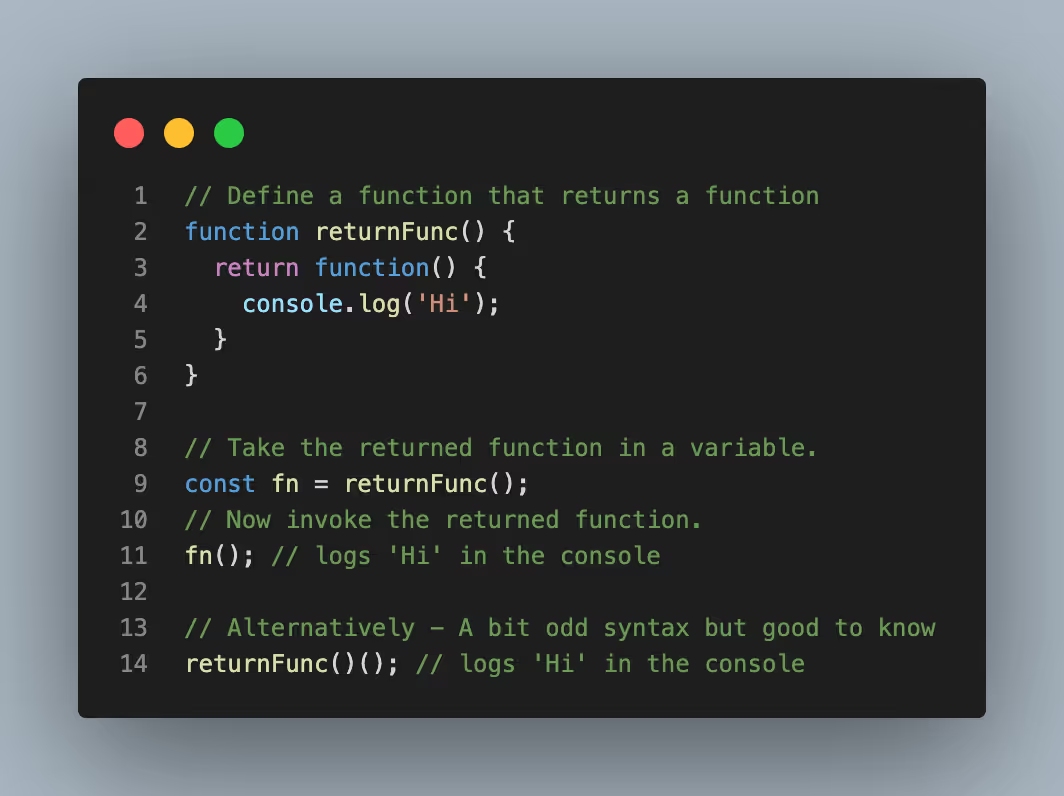
The most interesting part of using higher-order functions is composition. Composition within the given context is a software engineering pattern, so take it easy! Just kidding, we simply write small functions that handle one piece of business logic. Then, we can compose complex functions by using the different small functions we have previously created. This reduces bugs in our codebase and makes our code much easier to read and understand.
JavaScript has some of these functions already built in. Some examples of higher-order functions are the following: Array.prototype.map(), Array.prototype.forEach(), Array.prototype.reduce() and others. Simple clever, right?
So many higher-order functions are already built into JavaScript, and you can even make your own ones.
Too much theory alert!
One way to use a HOC is a conditional rendering helper. Let’s imagine you have a List component that should render a list of items or nothing if the list item is empty or null.
The HOC component is a great choice here, since it should shield away null or empty values or render nothing when there is no list. On the other hand, the plain List component should no longer worry about a non-existent list anymore, as it only cares about rendering the list. HOCs are commonly used to design components with certain shared behaviour in a way that makes them connected differently than normal state-to-props pattern
Using with is a useful convention to prefix a HOC, while JavaScript ES6 would allow expressing the HOC better with an ES6 arrow function.

In this example, the input component stays the same as the output, so nothing happens. It is possible to improve this essential functionality by introducing the loading state check to show the Loading component when the state is true or otherwise display the input component. A conditional rendering is a great use case for a HOC.
A conditional rendering is another great HOC usage. In general, it can be very efficient to spread an object as the props object in the previous example as input for a component. See the difference in the following code snippet:
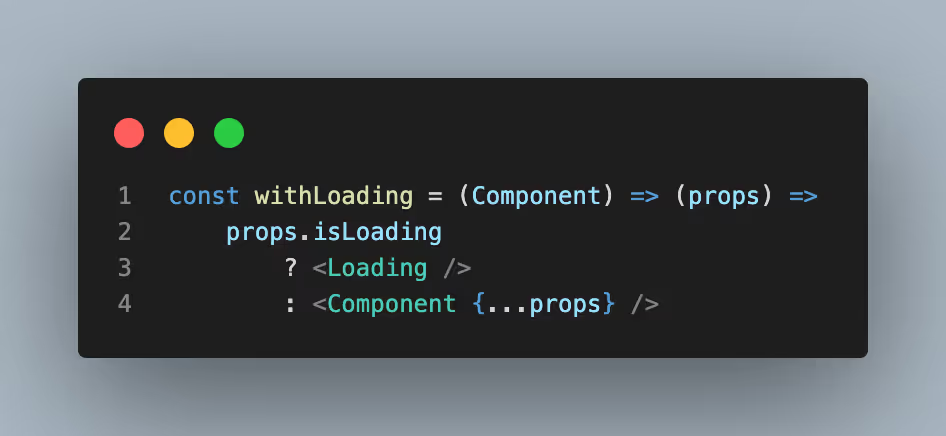
We passed all the props including the isLoading property by spreading the object into the input component. The input component may not care about the isLoading property. You can use the ES6 rest destructuring to avoid it:
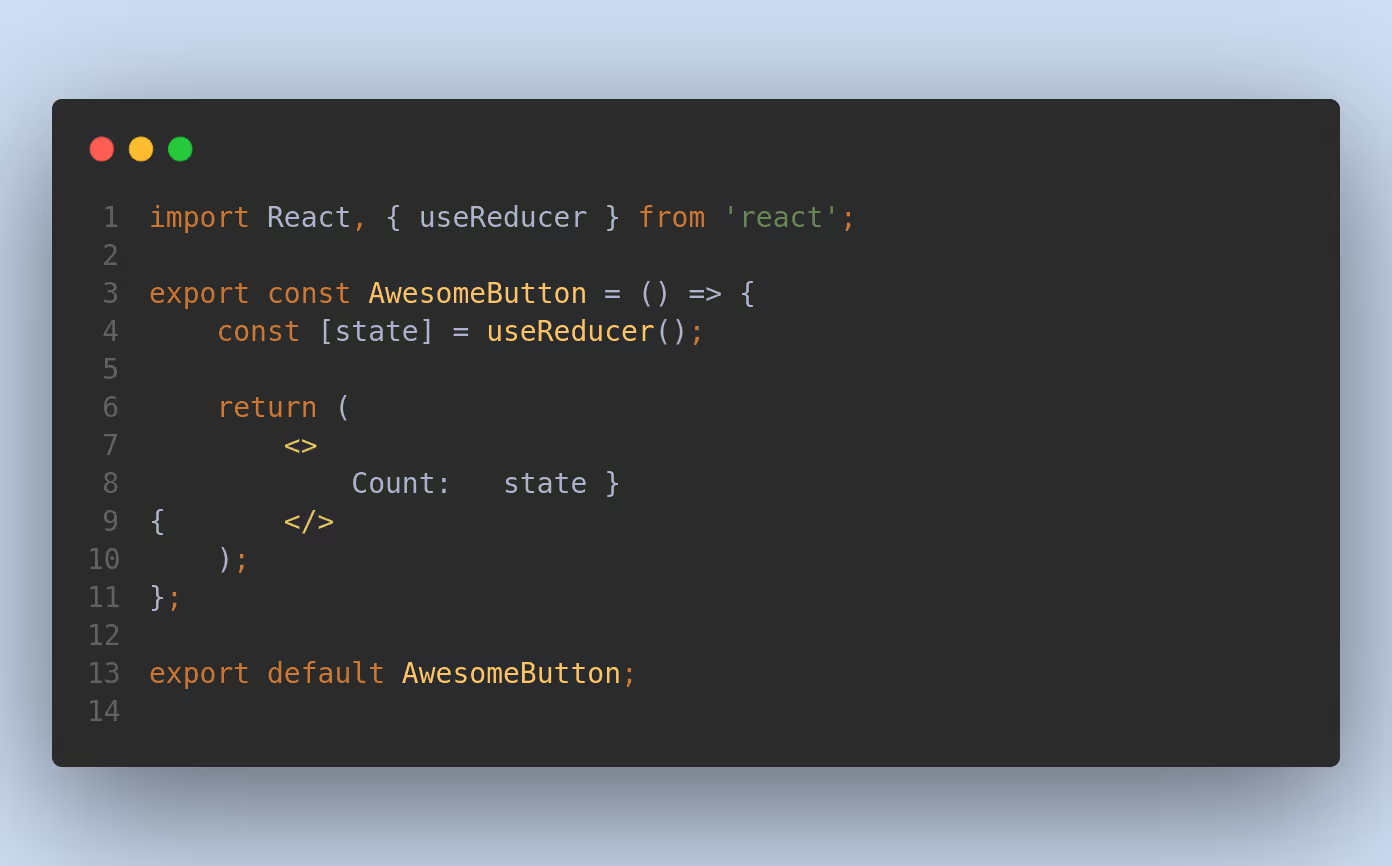
It takes one property out of the object but keeps the remaining object, which also works with multiple properties. More can be read about it in Mozilla’s destructuring assignment. Now you can use the HOC in JSX.
How about implementing the “Show more” button or the Loading component? The Loading component is already encapsulated in the HOC, but the input component is still missing. For showing either a Button component or a Loading component, the Button is the input component of the HOC. The enhanced output component is a ButtonWithLoading component.

As the last step, you have to use the ButtonWithLoading component, which receives the loading state as an additional property. While the HOC consumes the loading property, all other props get passed to the Button component.
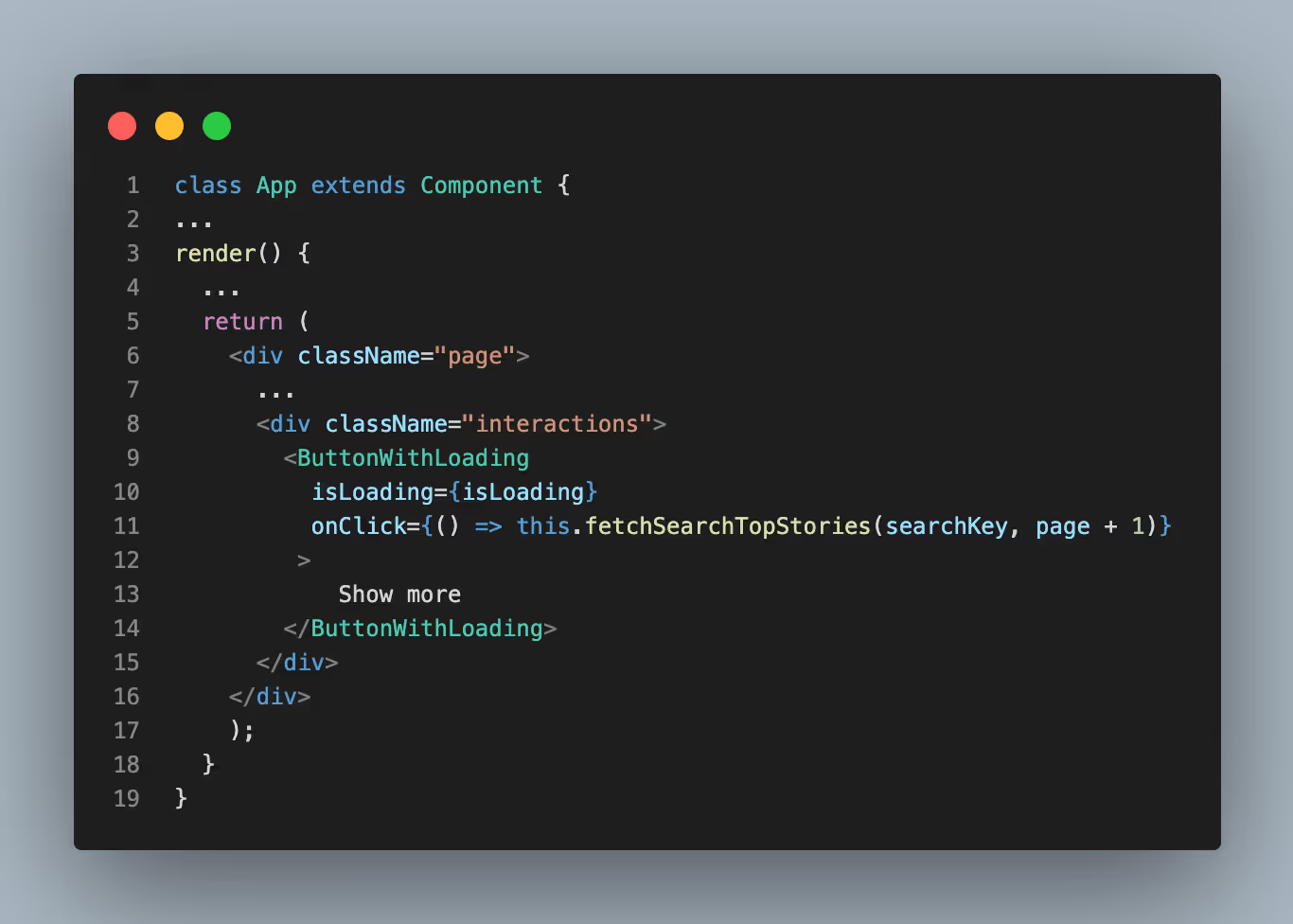
Higher-order components are an advanced pattern in React. They have multiple purposes:
- Improved component reusability
- Greater abstraction
- Components composability
- Props, state and view manipulations
This guide is a gentle introduction to higher-order components. It enables you another approach to learning them, shows an elegant way to use them in a functional programming way, and solves the problem of conditional rendering.
You can now improve your skills and practically wrap your head around by playing with the HOC within the external sandbox.